Validate email address in PHP
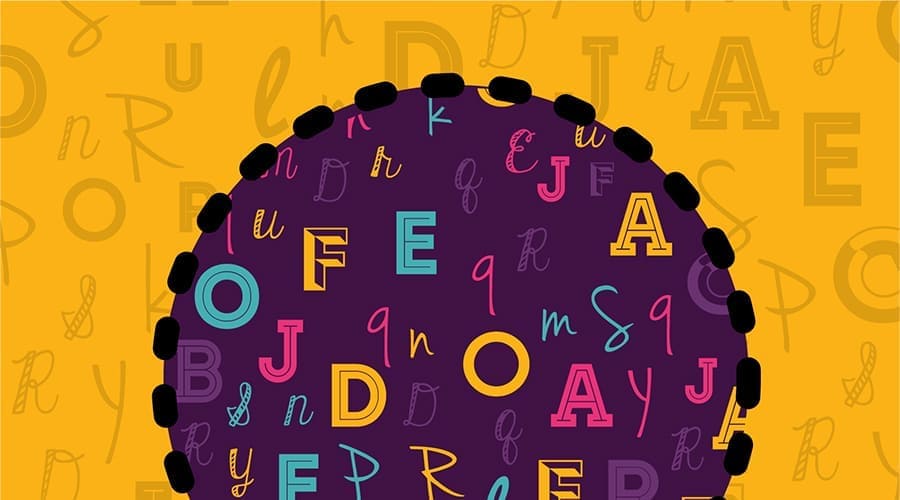
Imagine you are coding a new PHP project and you realize you need to validate email addresses e.g. from sign-up or newsletter form.
Fortunately PHP comes with very handy function filter_var which is available since PHP version 5.2.0. In general, this validates email addresses against the addr-spec syntax in RFC 822, with the exceptions that comments and whitespace folding and dotless domain names are not supported.
Ok, let's see how to validate email address format in a real example:
$email = $_POST["email"];
if (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo "Invalid email format";
//do your stuff here
}
Pretty simple, huh? On the other hand this code only verifies if the email address is syntactically correct. In case of registration the good practice is to send a verification email to the user immediately after signup.
You can also verify user's email address with our simple email validation API where you get 99% sure the email is valid and deliverable.