How to Use jQuery to Validate an Email Address
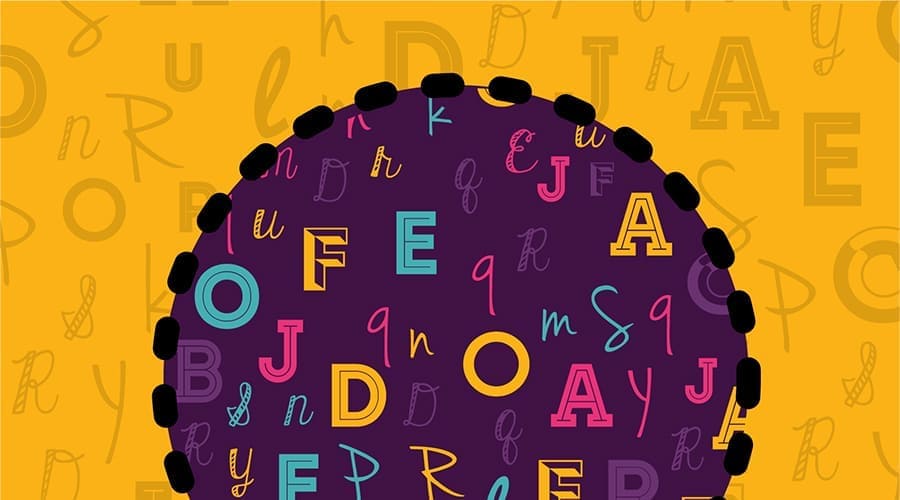
Simple validation using basic jQuery
Are you working on a web project and need to make sure users are entering a valid email address? jQuery, a popular JavaScript library, makes this task simple and efficient. This article will guide you through using jQuery to validate email addresses, aimed at programmers with basic skills.
While jQuery validation, included with plugins, effectively checks email syntax, it doesn't verify the email's actual existence or deliverability, making further email verification steps essential for ensuring user data quality and communication success. Try SendBridge email verification service and get 300 validations for free!
Now let's continue with our guide.
First, ensure jQuery is added to your project. You can include it by adding the following script tag in the <head>
section of your HTML:
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
Writing the Validation Code
With jQuery loaded, we can write a function to check if an email address is valid. The idea is to use a regular expression (regex) to test the email format. Don't worry; you don't need to know how to write this regex. We'll provide it for you.
Here's a simple jQuery function that does the job:
function validateEmail(email) {
var emailReg = /^([\w-\.]+@([\w-]+\.)+[\w-]{2,4})?$/;
return emailReg.test(email);
}
This function takes an email address as input and returns true
if the email matches the regex pattern, indicating it's valid. Otherwise, it returns false
.
Putting It Into Action
To use this function, you need to capture the user's input and pass it to validateEmail()
. You can do this by adding an event listener to the input field where users type their email addresses.
Assuming your email input field has an ID of "emailInput", here's how you can do it:
$(document).ready(function() {
$('#emailInput').on('blur', function() {
var email = $(this).val();
if(validateEmail(email)) {
alert('Email is valid!');
} else {
alert('Invalid Email Address');
}
});
});
This code listens for a "blur" event, which occurs when the input field loses focus (for example, when the user clicks away from it). It then grabs the value from the input, checks it with our validateEmail
function, and alerts the user whether their email is valid or not.
Improving User Experience
Alerts can be a bit jarring. For a better user experience, consider showing a message right on the form. You can do this by adding a small <span>
tag near your email input and updating its content based on the validation result:
<input type="text" id="emailInput" placeholder="Enter your email">
<span id="emailFeedback"></span>
Then, update your jQuery code to change the text of #emailFeedback
instead of using alert()
:
$(document).ready(function() {
$('#emailInput').on('blur', function() {
var email = $(this).val();
if(validateEmail(email)) {
$('#emailFeedback').text('Email is valid').css('color', 'green');
} else {
$('#emailFeedback').text('Invalid Email Address').css('color', 'red');
}
});
});
Now, when the user enters their email, they'll immediately see a helpful message indicating whether it's valid.
Using jQuery to validate email addresses is a straightforward process that greatly improves the user experience on your website. By including the jQuery library, writing a simple validation function, and applying it to user input, you can ensure that users provide valid email addresses in a user-friendly way. Remember to always enhance the user experience by providing clear and immediate feedback on their input. Happy coding!
Email Validation with the jQuery Validate Plugin
In the first part of our guide, we covered how to validate email addresses using basic jQuery. Now, let's take our validation to the next level with the jQuery Validate plugin. This powerful plugin simplifies form validation, including email addresses, making your code cleaner and more efficient.
Setting Up jQuery Validate
Before we start, make sure you've included both jQuery and the jQuery Validate plugin in your project. Add these script tags in your HTML file's <head>
section:
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-validate/1.19.3/jquery.validate.min.js"></script>
Basic Email Validation with jQuery Validate
jQuery Validate makes it super easy to ensure your users are entering valid email addresses. First, ensure your form and email input field are properly set up. Here's a simple example:
<form id="myForm">
<input type="email" name="email" id="emailInput" required>
<button type="submit">Submit</button>
</form>
Notice the type="email"
attribute in the input field. This tells the browser (and jQuery Validate) that this field should contain an email address.
Now, let's use jQuery Validate to check the form:
$(document).ready(function() {
$("#myForm").validate({
rules: {
email: {
required: true,
email: true
}
},
messages: {
email: {
required: "Please enter your email address",
email: "Please enter a valid email address"
}
}
});
});
In this code, we call the .validate()
method on our form. We define rules for our inputs, in this case, email
. We specify that the email is required
and must be a valid email (email: true
). We also set custom messages to display for each rule when it's not met.
Advanced Features
The jQuery Validate plugin offers much more than basic validation. You can customize it extensively to fit your needs. For example, you can add custom validation methods if you need to check something unique:
$.validator.addMethod("domain", function(value, element) {
return this.optional(element) || /^[\w-\.]+@example\.com$/.test(value);
}, "Please enter an email address with the domain @example.com");
$("#myForm").validate({
rules: {
email: {
required: true,
email: true,
domain: true // Use our custom validation method
}
}
});
This adds a new validation method called "domain" that checks if the email address belongs to "example.com". It's a great way to enforce email addresses from a specific domain.
Improving User Feedback
The jQuery Validate plugin automatically handles displaying error messages, but you can customize this behavior. For example, you can specify where and how to display these messages, or use highlight and unhighlight functions to add or remove error classes:
$("#myForm").validate({
// Validation rules...
highlight: function(element) {
$(element).addClass('error');
},
unhighlight: function(element) {
$(element).removeClass('error');
}
});
This will add an 'error' class to fields with validation errors, and remove it when the error is corrected. It's a simple way to visually indicate which fields need attention.
The jQuery Validate plugin is a powerful tool for ensuring your forms are filled out correctly, making your site more user-friendly and your data more reliable. By combining basic validation rules with advanced features and custom methods, you can create a robust validation system that meets your specific needs. Remember, good validation is key to a great user experience, so take advantage of these tools to create the best experience for your users. Happy coding!