Regular expression (regex) for email validation
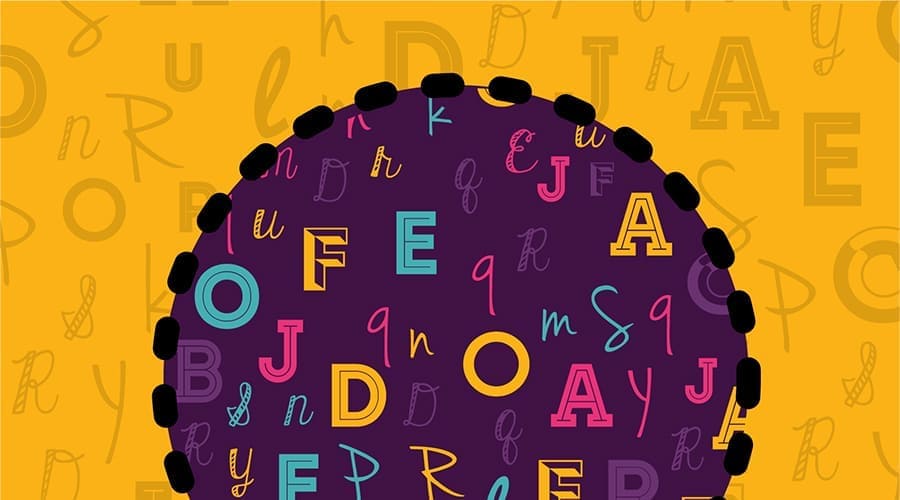
Regular expressions (regex) for email validation in some of the most common programming languages:
JavaScript:
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
Python:
import re
emailRegex = re.compile(r'^[^\s@]+@[^\s@]+\.[^\s@]+$')
PHP:
$emailRegex = '/^[^\s@]+@[^\s@]+\.[^\s@]+$/';
Java:
import java.util.regex.Pattern;
Pattern emailRegex = Pattern.compile("^[^\\s@]+@[^\\s@]+\\.[^\\s@]+$");
C#:
using System.Text.RegularExpressions;
Regex emailRegex = new Regex(@"^[^\s@]+@[^\s@]+\.[^\s@]+$");
These regex patterns will match most valid email addresses and can be used in the respective programming languages for email validation. Note that there are many variations of email addresses that can be considered valid, and these patterns may not match all of them. Additionally, it's always important to consider additional validation methods, such as sending a confirmation email to the address provided, to ensure the email is valid and belongs to the user.