Master Regex for JavaScript Email Validation
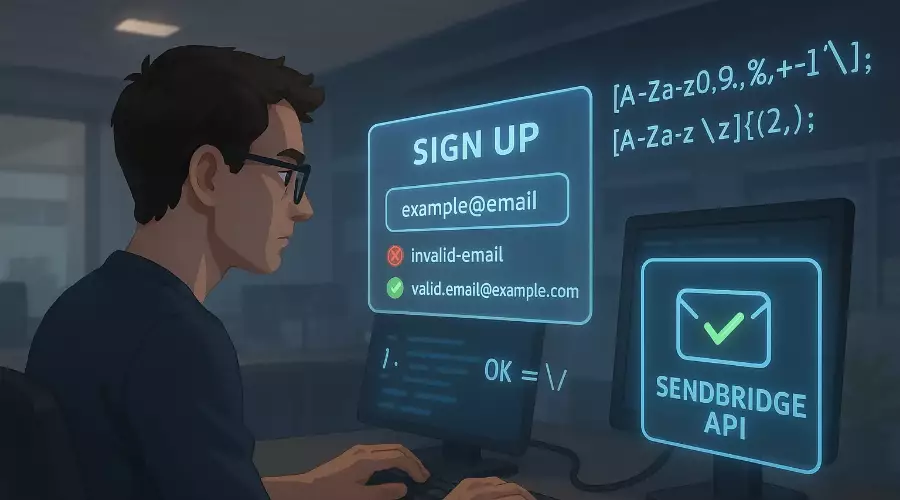
Email validation is a fundamental aspect of web development that ensures users submit correct information when signing up for services or communicating online. As a developer, ensuring that your forms capture valid email addresses can save you from headaches such as invalid signups, spam, or misdirected messages. With JavaScript, you can employ regular expressions (regex) to create efficient and straightforward validation logic right in your client-side code.
Beyond development convenience, validating email addresses helps maintain a cleaner database and reduces the risk of sending messages to addresses that simply do not exist. This practice also strengthens your relationship with email service providers, as you are less likely to be flagged for sending unwanted emails. Whether you're creating a simple sign-up form or a robust user management system, implementing reliable email validation logic should be high on your priority list.
Why Email Validation Matters
A single invalid address can disrupt your communication strategy and tarnish your sender reputation. If an email bounces frequently, your messages might be marked as spam, causing legitimate emails to land in the junk folder. This is where specialized tools like Sendbridge step in. Sendbridge verifies real email existence and ensures your mailing list remains accurate, thus preventing wasted efforts on non-existent addresses. Whether you're verifying one email or thousands in batches, Sendbridge offers a streamlined approach to quality control.
Beyond preventing errors, validated emails enhance user experience. Consider an e-commerce platform where customers expect receipts and shipping updates. Incorrect addresses mean missed alerts, user frustration, and potential revenue loss. When you validate user inputs with JavaScript's built-in methods or regular expressions, you minimize these risks and maintain higher satisfaction levels.
Understanding Regular Expressions
Regular expressions are patterns that help match character combinations in strings, making them an ideal fit for filtering out invalid emails. By defining a pattern that captures the general structure of an email address, you can quickly confirm whether the user input meets basic formatting requirements. Common regex usage involves checking for symbols like the "@” sign, valid domain formats, and top-level domains, although the patterns can get quite advanced.
In simpler terms, a regex can be seen as a specialized search tool that looks for the exact formation of an email. For example, a typical pattern will ensure there is text before and after the "@” symbol, and that there is a period somewhere after the "@” to signify the domain extension. While some developers shy away from regex due to perceived complexity, a basic understanding can go a long way in crafting robust JavaScript validation.
Crafting a Basic Regex Pattern
A simple JavaScript regex for email validation might look something like this:
/^[^\s@]+@[^\s@]+\.[^\s@]+$/
This pattern checks for characters that are not spaces or "@” symbols, ensures an "@” is present, and looks for at least one period after the "@”. Although this covers the most common cases, it may not account for every possible edge scenario, such as lengthy domain extensions, plus signs in email addresses, or special characters.
Step-by-Step Validation Logic
To keep your validation logic organized, create a dedicated function in your JavaScript code. For example, let's define a function called validateEmail(email). Within this function, apply the regex and return true if it matches or false otherwise. This approach isolates your validation logic, enabling you to reuse it across multiple forms or parts of your application.
Example of Basic Validation Function
function validateEmail(email) {
const regex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return regex.test(email);
}
By using regex.test(email)
, you can quickly confirm if the user input adheres to the specified pattern. If it does, the function returns true; otherwise, it returns false. This modular setup allows you to call validateEmail wherever needed, whether it's during form submission or in real-time input validation.
Extended Email Validation Example
Here's an example of a professional and extended email validation function in JavaScript. It improves on the basic example by including more comprehensive checks, such as trimming whitespace, providing error messages, and allowing for optional configuration.
/**
* Validates an email address with extended rules and customizable options.
*
* @param {string} email - The email address to validate.
* @param {Object} [options] - Optional settings.
* @param {boolean} [options.allowSubdomains=true] - Whether subdomains are allowed.
* @returns {{ valid: boolean, error?: string }}
*/
function validateEmailAdvanced(email, options = {}) {
const { allowSubdomains = true } = options;
if (typeof email !== 'string') {
return { valid: false, error: 'Email must be a string.' };
}
const trimmed = email.trim();
if (trimmed.length === 0) {
return { valid: false, error: 'Email cannot be empty.' };
}
// Basic RFC 5322-compliant pattern (simplified but more robust)
const basicPattern = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
if (!basicPattern.test(trimmed)) {
return { valid: false, error: 'Email format is invalid.' };
}
// Optional subdomain restriction
if (!allowSubdomains) {
const domainParts = trimmed.split('@')[1].split('.');
if (domainParts.length > 2) {
return { valid: false, error: 'Subdomains are not allowed.' };
}
}
return { valid: true };
}
Usage:
const email = "user@example.com";
const result = validateEmailAdvanced(email);
if (result.valid) {
console.log("Email is valid.");
} else {
console.warn("Invalid email:", result.error);
}
This approach is more suitable for production use because it:
- Trims input
- Provides specific feedback
- Allows you to configure rules (e.g., subdomain handling)
- Returns a result object rather than a plain boolean — useful for UI validation
Real-Life Use Cases
Imagine you run an online newsletter that delivers daily coding tips and industry updates. If your sign-up form lacks proper email validation, many addresses will inevitably bounce. By integrating a function like validateEmail and using advanced verification tools such as Sendbridge, you can ensure that only legitimate subscribers join your mailing list. This not only preserves your sender reputation but also provides a more engaging experience for your audience.
Another scenario is registering accounts in a SaaS platform, where incorrect email addresses can lead to missed password resets or critical system notifications. A robust validation script mitigates these concerns. By checking each input field against a well-structured regex, you minimize data entry errors. For additional assurance, you can leverage Sendbridge for bulk verification, especially useful when you have a large database to clean up or maintain.
Best Practices for Enhanced Validation
When crafting your regex patterns, remember to accommodate different domain formats. For instance, some businesses use country code top-level domains (TLDs) like ".uk” or ".au,” and ignoring them might invalidate legitimate users. Additionally, consider permitting commonly used characters like plus "+" or underscores "_" in the local-part of the email. Striking the right balance between strictness and flexibility is key to a seamless user experience.
One best practice is to pair client-side validation with server-side checks. JavaScript validation improves user experience by catching errors quickly, but it is not foolproof. A malicious actor can bypass client-side checks by disabling JavaScript or sending data directly to your backend. Therefore, always validate data on the server before processing it further. Tools like Sendbridge can serve as an added layer of defense by confirming that the address indeed exists.
Common Pitfalls to Avoid
Relying solely on a single regex pattern without considering the range of legitimate email formats is a frequent error. Some providers allow longer domain names or special characters that your pattern might miss. Additionally, placing overly restrictive rules—such as prohibiting all characters except letters or digits—can frustrate users with valid addresses. Being aware of these pitfalls can save you from unexpected bounce rates or user complaints.
Bullet List of Helpful Pointers
- Start with a solid yet flexible regex to handle special characters, subdomains, and country-code TLDs.
- Always use server-side validation in tandem with JavaScript checks for reinforced data integrity.
- Consider specialized tools like Sendbridge to verify the real existence of an email address for higher accuracy.
- Regularly update your validation logic as new TLDs emerge and email providers introduce new formats.
Leveraging Industry Resources
If you're looking to broaden your regex expertise, you can consult Mozilla Developer Network (MDN), which provides extensive documentation and examples to help developers understand and implement regex patterns effectively. Additionally, services like Sendbridge offer dedicated APIs and features that integrate well with your existing JavaScript workflows. For in-depth learning or troubleshooting, there are also numerous forums, courses, and online communities ready to provide assistance.
Integrating External Libraries and Services
To further streamline your JavaScript projects, you might also consider libraries such as validator.js. This popular utility offers a range of validation functions, including email checks, to simplify your code. Nevertheless, even the most comprehensive library cannot guarantee that the email address actually exists. This is why integrating an email verification service such as Sendbridge can offer an extra layer of certainty.
In a real-world setting, consider a scenario where you've built a vibrant e-commerce platform that automatically sends order confirmations, shipping updates, and discount coupons. If any of these emails bounce, your marketing strategy and customer service can suffer. By pairing a reputable library like validator.js with Sendbridge, you'll gain both syntactical and existential validation, ensuring each piece of communication actually reaches its intended recipient.
Reaching Higher Efficiency with Batch Verification
When your platform scales, verifying emails one by one can quickly become inefficient. That's why batch verification is incredibly valuable for high-volume operations. Sendbridge excels here, allowing you to upload or submit lists of addresses for a comprehensive check. Imagine running a monthly newsletter with tens of thousands of subscribers. With just a quick batch verification, you eliminate stale or invalid entries and maintain a healthy sender reputation, which is essential for open rates and user engagement.
Learning Resources and JavaScript Assignment Help
For anyone aspiring to deepen their JavaScript knowledge, or even if you're stuck with a specific project, specialized assistance can be immensely beneficial. If you're struggling with email validation or any other coding issues, you might find dedicated support through JavaScript assignment help. Learning from experienced mentors not only saves time but also strengthens your overall coding fundamentals. This way, you can focus on building dynamic features and robust forms without sacrificing quality or security.
Smarter Email Validation Strategies
Email validation in JavaScript is a key part of maintaining clean data and effective communication. Leveraging regular expressions for client-side checks offers quick feedback to users, while server-side validation adds an essential layer of security. When paired with tools like Sendbridge, your workflow becomes both efficient and robust—enhancing user trust and preserving your sender reputation over time.