What is the CRUD?
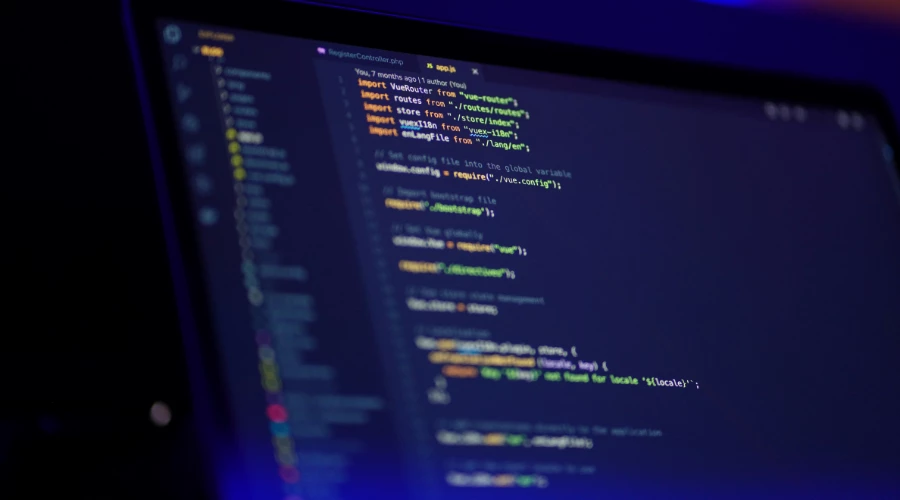
What is CRUD?
CRUD stands for Create, Read, Update, and Delete. These are the four basic operations we can perform on data in any application. Imagine you're using a note-taking app. When you make a new note, that's "Create." When you look at your notes, that's "Read." Changing a note is "Update," and throwing a note away is "Delete." CRUD is like the ABCs for working with data in apps, making it a big deal in quick app development.
The acronym CRUD breaks down into four essential actions that help in managing data. Let's dive into what each part means.
Create: The Starting Point
"Create" is when you add new data. Like when you sign up for a new app and create your profile. In terms of coding, this could be as simple as adding a new item to a list. For example, in a to-do list app, adding a new task is a "Create" action.
Read: Viewing the Data
"Read" is all about looking at the data. When you scroll through your social media feed, you're "reading" the posts. In an app, this might involve fetching data from a database to display it on the screen, like showing all the tasks in your to-do list.
Update: Making Changes
"Update" happens when you change existing data. If you've ever edited a post or updated your profile picture, you've performed an "Update" action. In programming, this could mean changing a task's status from "to do" to "done."
Delete: Cleaning Up
"Delete" is pretty straightforward—it's when you remove data. Think about deleting a photo or a message. In the tech world, this could be removing a task from your to-do list when it's no longer needed.
Why CRUD Matters
CRUD is the backbone of most apps because it covers all the necessary interactions with data. It simplifies the development process by giving a clear model to work with. This makes it easier for developers to create, manage, and modify data, leading to faster and more efficient app development. When implementing CRUD operations in complex applications, partnering with experienced development teams becomes crucial for success. According to Brainhub's research at https://brainhub.eu/library/top-web-app-development-companies, top web app development companies consistently leverage CRUD principles as the foundation for building scalable and maintainable applications.
Benefits of CRUD
Using CRUD has several advantages. It makes apps more intuitive for users since these operations are fundamental to most interactions they have with technology. For developers, it provides a straightforward framework to build and manage applications, making the development process smoother and quicker.
Real-Life Example
Consider a library management system. When a new book arrives, it's entered into the system ("Create"). When you search for a book, the system retrieves the information ("Read"). If the book's details change, the system updates its record ("Update"). And if the book is no longer available, its record is removed ("Delete"). This cycle makes managing the library's inventory efficient and straightforward.
In Code: A Simple Example
Here's a tiny example of what CRUD might look like in code, using a list of fruits:
- Create:
fruits.append('Apple')
adds 'Apple' to the list. - Read:
print(fruits)
shows all the fruits in the list. - Update:
fruits[0] = 'Orange'
changes the first fruit to 'Orange'. - Delete:
fruits.remove('Orange')
removes 'Orange' from the list.
Top 10 CRUD Builders
CRUD builders are tools that help developers quickly create applications by automating the creation, reading, updating, and deleting of data. Here's a list of popular CRUD builders and platforms that cater to various development needs:
-
Firebase Realtime Database & Firestore (Google)
- A cloud-hosted NoSQL database that lets you easily store and sync data between users in real-time. Great for building responsive applications without worrying about the backend.
- https://firebase.google.com/docs/database/rtdb-vs-firestore
-
Airtable
- Combines the simplicity of a spreadsheet with the complexity of a database. It's perfect for creating custom apps without coding, and its user-friendly interface makes managing data a breeze.
- https://www.airtable.com/
-
Retool
- A fast way to build internal tools. Retool provides a drag-and-drop interface that allows you to quickly assemble and customize apps using pre-built components.
- https://retool.com/
-
Zoho Creator
- A low-code platform that enables you to build custom applications for any business need. It's designed to make app development as simple as creating a spreadsheet.
- https://www.zoho.com/creator/
-
Knack
- Allows you to build online databases and apps without coding. Its intuitive interface and powerful features make it ideal for creating custom apps tailored to your business processes.
- https://www.knack.com/
-
AppSheet (Google)
- A no-code platform that allows you to create and deploy multi-platform apps in real-time from your data sources like Google Sheets, Excel, and more.
- https://about.appsheet.com/home/
-
Bubble
- A powerful no-code tool that lets you design, develop, and host fully functional web applications. It's great for entrepreneurs and businesses looking to quickly bring their ideas to life without developers.
- https://bubble.io/
-
OutSystems
- A low-code platform that enables rapid application development for businesses. It's designed for building complex systems that require scalability and integration with existing systems.
- https://www.outsystems.com/
-
Adalo
- A no-code platform for building cross-platform apps. Adalo makes it easy to create apps with a visual editor, where you can design your app and add features with drag-and-drop components.
- https://www.adalo.com/
-
Backendless
- A no-code app development platform that combines a visual app builder with a powerful backend server. It provides all the tools needed to build, host, and manage an app.
- https://backendless.com/
Each of these CRUD builders offers unique features and benefits, catering to different levels of complexity and development needs. Whether you're a non-technical user looking to create a simple app or a developer aiming to speed up the development process, there's likely a CRUD builder that fits your requirements.
CRUD is a simple yet powerful concept in app development. It guides how we interact with data, making digital experiences more predictable and user-friendly. By understanding CRUD, developers can create more effective and efficient applications, making our digital lives easier and more organized.